Introduction to composer – PHP package manager
January 30, 2015
I. What is composer
Composer is a tool for dependency management in PHP. It allows you to declare the dependent libraries your project needs and it will install them in your project for you – Official Website
II. Normal routine with Silex and PHPUnit
Silex is the most popular PHP micro-framework.
We will use it with PHPUnit to show how fun and easy Composer work, with TDD Style 😀
Hello world
a. Require silex micro-framework
$ composer require silex/silex:~1.2
This command will also create composer.json file for the first time
This file is where we config required packages, namespace rules (PSR-0/PSR-4 style), binary scripts, hook scripts… Yes, composer can do a lot, and so important for continuous integration environment also!
After this test, our folder structure should be like this:
-rw-rw-r-- 1 tuannh tuannh 154 Th01 29 20:13 composer.json -rw-rw-r-- 1 tuannh tuannh 51087 Th01 29 20:14 composer.lock drwxrwxr-x 11 tuannh tuannh 4096 Th01 29 20:14 vendor
Note that vendor/ folder is where our packages places. This should be ignored from your VCS (Git, SVN..)
And also composer.lock is generated. This magic file is where composer store the “exact version” of our packages. This is very important to keep team members, and production machines stay on the SAME version (even commit hash) of library. This is to avoid the situation where we have same version v1.2 of silex BUT different commit hash, which could make difference somehow.
b. Functional Test (TDD Style)
Well, OK. Let’s write a functional test case, like a boss!
$ composer require symfony/browser-kit
We will use ‘browser-kit’ package, a component from symfony, to write test
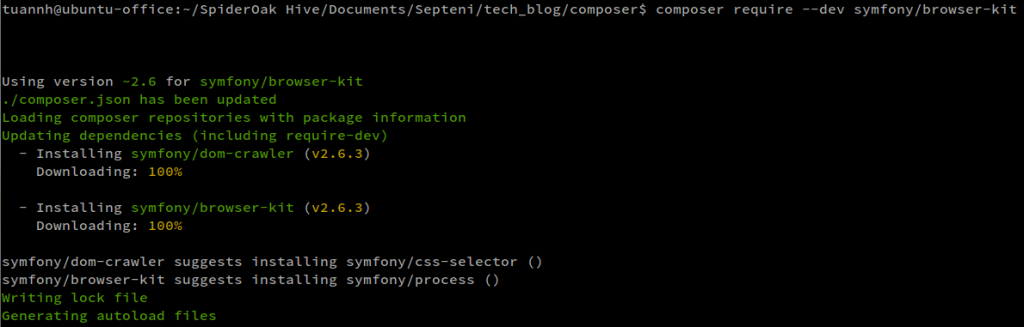
The final composer.json file can be found here
We’d expect a Hello World test case
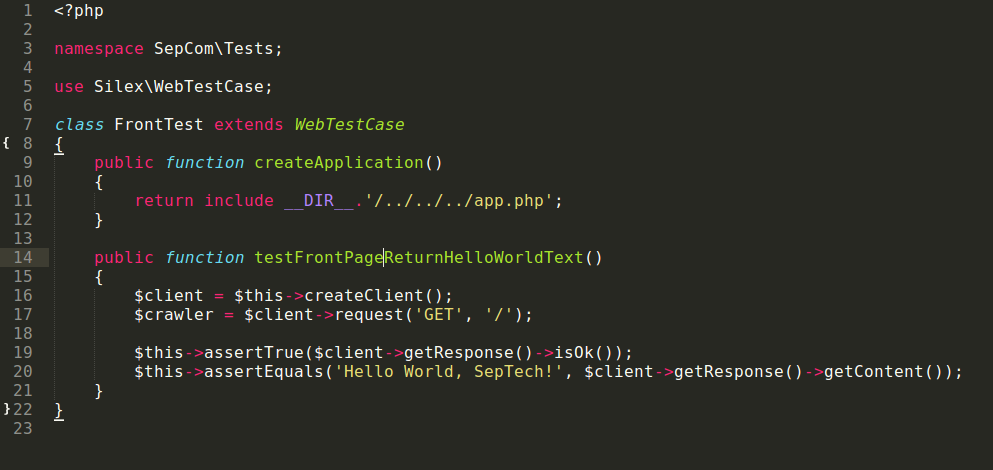
The app.php in the above test code is the bootstrap file, where we init the SilexApplication:
<?php require_once __DIR__.'/vendor/autoload.php'; // Init the Silex Application $app = new Silex\Application(); // Well, we're debugging $app['debug'] = true; // Return the App object, which will be use for Production Application and Test return $app;
Of course, the red color will be seen
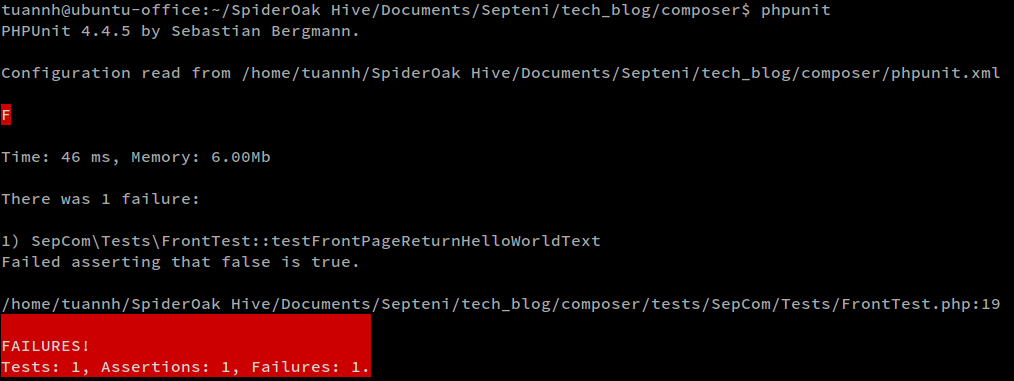
c. Production code here
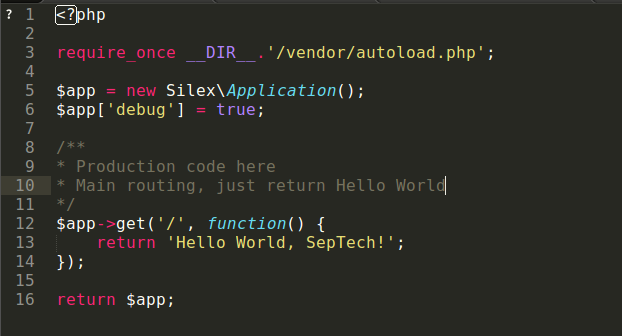
Green color!
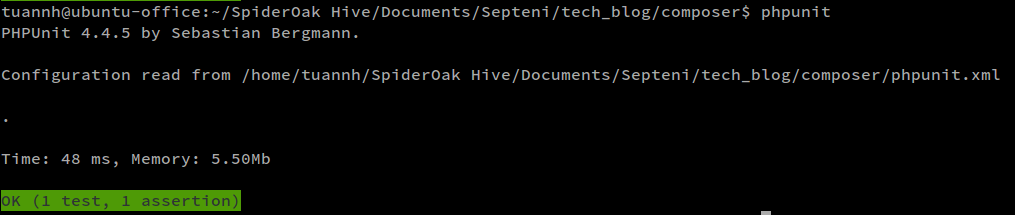
After a little setup with your webserver of choice (nginx/apache), we can see the webpage run well!
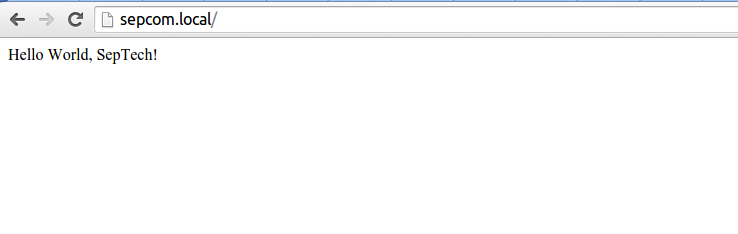
III. Packagist (https://packagist.org/)
It’s a Composer repository, where we can search/submit any composer-compliant packages, and even find useful information of those packages, such as how much downloads they got, how many stars people worldwide rated them
For example some popular PHP packages
– Logging: Monolog
– Email: Swift Mailer
– Database migration: Phinx
– Database Layer: Doctrine
– Crawler: Guzzle
IV. Notes
– Push and store composer.json vs composer.lock with your VCS of choice (Git, SVN, Perfoce…)
– Don’t run `composer update`, without any specific packages, unless you know what you’re doing
– Use option Prefer-dist (instead of prefer-source as default) whenever you can. It can speed up the composer installation/update a lot.
– require-dev vs require:
require-dev is where we define packages needed for development/building phase, but will not play any role on production code. For example: phpunit (for testing), phpmd (for coding convention checking)…
Be ready for production
Just a reminder, before deploying your code in production, don’t forget to optimize the autoloader:
$ composer dump-autoload --optimize
This can also be used while installing packages with the --optimize-autoloader
option. Without that optimization, you may notice a performance loss from 20 to 25%.
Conclusion
Source code can be found here: https://github.com/septech/silex-functional-test